前言:JUC包,ReentrantLock源码学习
简介
ReentrantLock实现Lock接口,内部类继承了AQS,是一种可重入的独占锁,支持公平锁和非公平锁两种方式。
常用方法
lock():获取锁,获取不到会等待
unlock():释放锁
ReentrantLock(boolean):构造方法,布尔参数决定公平或不公平,默认false
类图
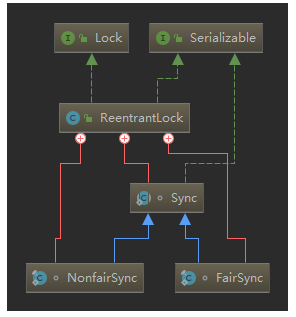
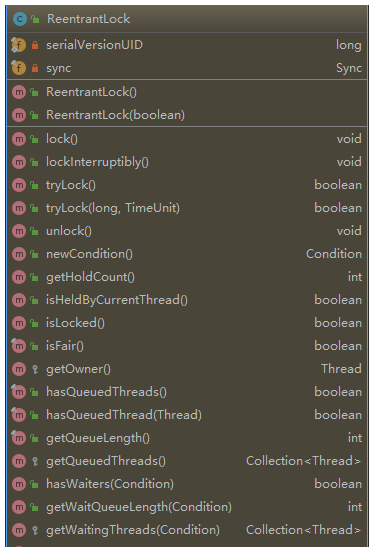
简单应用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
|
public class MyThread implements Runnable{ ReentrantLock lock = new ReentrantLock(); @Override public void run() { lock.lock(); System.out.println(Thread.currentThread().getName()+"获得锁"); try { Thread.sleep(10); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(Thread.currentThread().getName()+"释放锁"); lock.unlock(); } }
public class ReentrantLockTest {
public static void main(String[] args) { MyThread myThead = new MyThread(); for(int i=0;i<100;i++){ new Thread(myThead).start(); } } }
|
源码学习
Sync
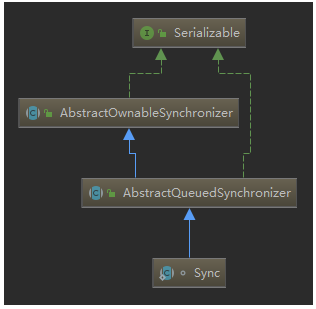
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
| abstract static class Sync extends AbstractQueuedSynchronizer { private static final long serialVersionUID = -5179523762034025860L;
abstract void lock(); final boolean nonfairTryAcquire(int acquires) { final Thread current = Thread.currentThread(); int c = getState(); if (c == 0) { if (compareAndSetState(0, acquires)) { setExclusiveOwnerThread(current); return true; } } else if (current == getExclusiveOwnerThread()) { int nextc = c + acquires; if (nextc < 0) throw new Error("Maximum lock count exceeded"); setState(nextc); return true; } return false; } protected final boolean tryRelease(int releases) { int c = getState() - releases; if (Thread.currentThread() != getExclusiveOwnerThread()) throw new IllegalMonitorStateException(); boolean free = false; if (c == 0) { free = true; setExclusiveOwnerThread(null); } setState(c); return free; } protected final boolean isHeldExclusively() { return getExclusiveOwnerThread() == Thread.currentThread(); } final ConditionObject newCondition() { return new ConditionObject(); } final Thread getOwner() { return getState() == 0 ? null : getExclusiveOwnerThread(); } final int getHoldCount() { return isHeldExclusively() ? getState() : 0; } final boolean isLocked() { return getState() != 0; } private void readObject(java.io.ObjectInputStream s) throws java.io.IOException, ClassNotFoundException { s.defaultReadObject(); setState(0); } }
|
NonfairSync
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| static final class NonfairSync extends Sync { private static final long serialVersionUID = 7316153563782823691L; final void lock() { if (compareAndSetState(0, 1)) setExclusiveOwnerThread(Thread.currentThread()); else acquire(1); } protected final boolean tryAcquire(int acquires) { return nonfairTryAcquire(acquires); } }
|
FairSync
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| static final class FairSync extends Sync { private static final long serialVersionUID = -3000897897090466540L; final void lock() { acquire(1); } protected final boolean tryAcquire(int acquires) { final Thread current = Thread.currentThread(); int c = getState(); if (c == 0) { if (!hasQueuedPredecessors() && compareAndSetState(0, acquires)) { setExclusiveOwnerThread(current); return true; } } else if (current == getExclusiveOwnerThread()) { int nextc = c + acquires; if (nextc < 0) throw new Error("Maximum lock count exceeded"); setState(nextc); return true; } return false; } }
|
构造方法
默认不公平模式
1 2 3 4 5 6 7
| public ReentrantLock() { sync = new NonfairSync(); }
public ReentrantLock(boolean fair) { sync = fair ? new FairSync() : new NonfairSync(); }
|
getHoldCount
返回加锁次数
1 2 3
| public int getHoldCount() { return sync.getHoldCount(); }
|
getOwner
返回获得锁的线程
1 2 3
| protected Thread getOwner() { return sync.getOwner(); }
|
getQueuedThreads
返回CLH队列的线程集合
1 2 3
| protected Collection<Thread> getQueuedThreads() { return sync.getQueuedThreads(); }
|
getQueueLength
返回CLH队列长度
1 2 3
| public final int getQueueLength() { return sync.getQueueLength(); }
|
getWaitingThreads
返回Condition队列的线程集合
1 2 3 4 5 6 7
| protected Collection<Thread> getWaitingThreads(Condition condition) { if (condition == null) throw new NullPointerException(); if (!(condition instanceof AbstractQueuedSynchronizer.ConditionObject)) throw new IllegalArgumentException("not owner"); return sync.getWaitingThreads((AbstractQueuedSynchronizer.ConditionObject)condition); }
|
getWaitQueueLength
返回Condition队列长度
1 2 3 4 5 6 7
| public int getWaitQueueLength(Condition condition) { if (condition == null) throw new NullPointerException(); if (!(condition instanceof AbstractQueuedSynchronizer.ConditionObject)) throw new IllegalArgumentException("not owner"); return sync.getWaitQueueLength((AbstractQueuedSynchronizer.ConditionObject)condition); }
|
hasQueuedThread
判断线程是否在CLH队列中
1 2 3
| public final boolean hasQueuedThread(Thread thread) { return sync.isQueued(thread); }
|
hasQueuedThreads
判断CLH队列是否有元素(线程)
1 2 3
| public final boolean hasQueuedThreads() { return sync.hasQueuedThreads(); }
|
hasWaiters
判断Condition队列是否有元素(线程)
1 2 3 4 5 6 7
| public boolean hasWaiters(Condition condition) { if (condition == null) throw new NullPointerException(); if (!(condition instanceof AbstractQueuedSynchronizer.ConditionObject)) throw new IllegalArgumentException("not owner"); return sync.hasWaiters((AbstractQueuedSynchronizer.ConditionObject)condition); }
|
isFair
判断是否公平锁
1 2 3
| public final boolean isFair() { return sync instanceof FairSync; }
|
isHeldByCurrentThread
判断当前线程是否获取到锁
1 2 3
| public boolean isHeldByCurrentThread() { return sync.isHeldExclusively(); }
|
isLocked
判断是否锁定
1 2 3
| public boolean isLocked() { return sync.isLocked(); }
|
lock
常用方法,获取锁
1 2 3
| public void lock() { sync.lock(); }
|
lockInterruptibly
同lock,可响应异常
1 2 3
| public void lockInterruptibly() throws InterruptedException { sync.acquireInterruptibly(1); }
|
newCondition
创建Condition对象
1 2 3
| public Condition newCondition() { return sync.newCondition(); }
|
toString
转化为String格式
1 2 3 4 5 6
| public String toString() { Thread o = sync.getOwner(); return super.toString() + ((o == null) ? "[Unlocked]" : "[Locked by thread " + o.getName() + "]"); }
|
tryLock
调用Sync的nonfairTryAcquire获取锁
1 2 3
| public boolean tryLock() { return sync.nonfairTryAcquire(1); }
|
限时的tryLock
1 2 3 4
| public boolean tryLock(long timeout, TimeUnit unit) throws InterruptedException { return sync.tryAcquireNanos(1, unit.toNanos(timeout)); }
|
unlock
释放锁
1 2 3
| public void unlock() { sync.release(1); }
|