前言:ArrayListSpliterator是ArrayList的静态内部类,本文将从源码分析与学习ArrayListSpliterator类
ArrayListSpliterator简介
ArrayListSpliterator实现了Spliterator接口,能并行遍历ArrayList集合,特征值为Spliterator.ORDERED | Spliterator.SIZED | Spliterator.SUBSIZED
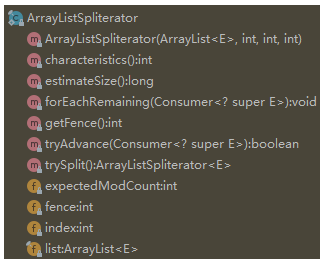
ArrayListSpliterator源码学习
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104
| static final class ArrayListSpliterator<E> implements Spliterator<E> { private final ArrayList<E> list; private int index; private int fence; private int expectedModCount; ArrayListSpliterator(ArrayList<E> list, int origin, int fence, int expectedModCount) { this.list = list; this.index = origin; this.fence = fence; this.expectedModCount = expectedModCount; }
private int getFence() { int hi; ArrayList<E> lst; if ((hi = fence) < 0) { if ((lst = list) == null) hi = fence = 0; else { expectedModCount = lst.modCount; hi = fence = lst.size; } } return hi; } public ArrayListSpliterator<E> trySplit() { int hi = getFence(), lo = index, mid = (lo + hi) >>> 1; return (lo >= mid) ? null : new ArrayListSpliterator<E>(list, lo, index = mid,expectedModCount); }
public boolean tryAdvance(Consumer<? super E> action) { if (action == null) throw new NullPointerException(); int hi = getFence(), i = index; if (i < hi) { index = i + 1; @SuppressWarnings("unchecked") E e = (E)list.elementData[i]; action.accept(e); if (list.modCount != expectedModCount) throw new ConcurrentModificationException(); return true; } return false; } public void forEachRemaining(Consumer<? super E> action) { int i, hi, mc; ArrayList<E> lst; Object[] a; if (action == null) throw new NullPointerException(); if ((lst = list) != null && (a = lst.elementData) != null) { if ((hi = fence) < 0) { mc = lst.modCount; hi = lst.size; } else mc = expectedModCount; if ((i = index) >= 0 && (index = hi) <= a.length) { for (; i < hi; ++i) { @SuppressWarnings("unchecked") E e = (E) a[i]; action.accept(e); } if (lst.modCount == mc) return; } } throw new ConcurrentModificationException(); } public long estimateSize() { return (long) (getFence() - index); }
public int characteristics() { return Spliterator.ORDERED | Spliterator.SIZED | Spliterator.SUBSIZED; } }
|
简单实例
list中存放26个字母,使用ArrayListSpliterator多线程并行遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class Main { public static void main(String[] args) { List<String> list = new ArrayList<>(); String letter = "abcdefghijklmnopqrstuvwxyz"; for (int i = 0; i < letter.length(); i++) { list.add(String.valueOf(letter.charAt(i))); } Spliterator<String> spliterator = list.spliterator(); System.out.println("使用estimateSize()估算大小:" + spliterator.estimateSize()); System.out.println("使用characteristics()获取特征值:" + spliterator.characteristics());
ExecutorService executorService = Executors.newFixedThreadPool(5); for(int i=0;i<4;i++){ executorService.execute(()->{ Spliterator temp = spliterator.trySplit(); temp.forEachRemaining(s -> System.out.println(Thread.currentThread().getName() + "输出list元素" + s)); }); }
executorService.execute(()->{ Spliterator temp = spliterator; temp.forEachRemaining(s -> System.out.println(Thread.currentThread().getName() + "输出list元素" + s)); }); executorService.shutdown(); } }
|
运行结果
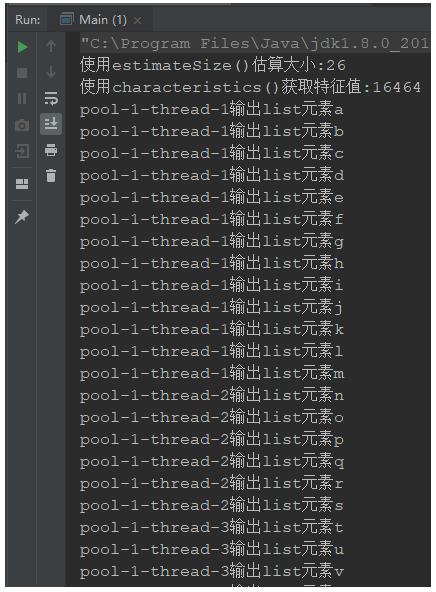